top of page
LENGUAJE DE PROGRAMACIÓN
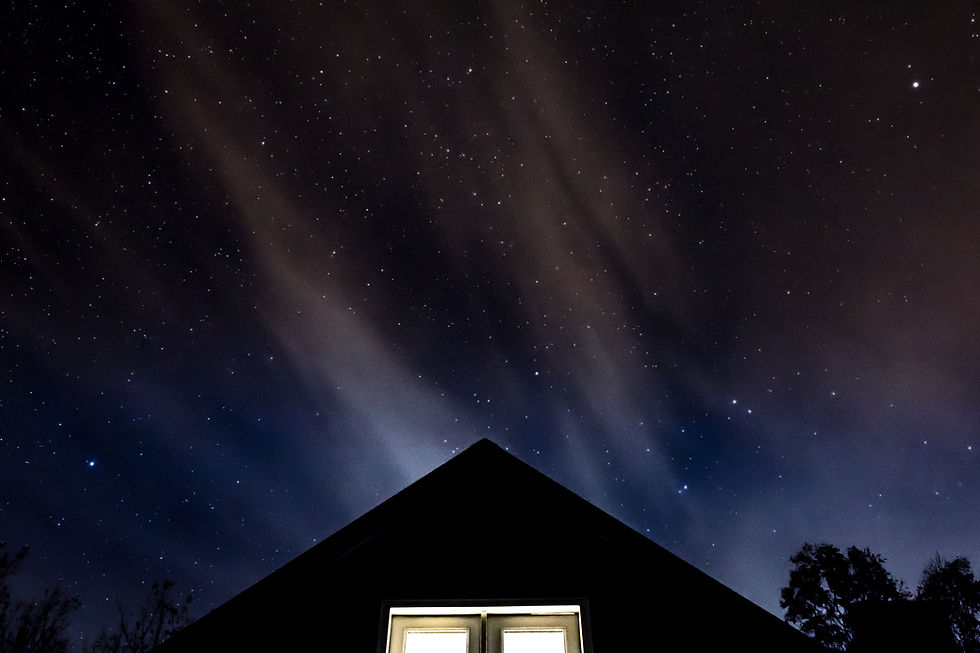
Matrices
Tarea hecha en casa
#include <iostream>
using namespace std;
const int MAX_FILAS = 3;
const int MAX_COLUMNAS = 3;
// Función para sumar dos matrices
void sumarMatrices(int matriz1[][MAX_COLUMNAS], int matriz2[][MAX_COLUMNAS], int resultado[][MAX_COLUMNAS]) {
for (int i = 0; i < MAX_FILAS; i++) {
for (int j = 0; j < MAX_COLUMNAS; j++) {
resultado[i][j] = matriz1[i][j] + matriz2[i][j];
}
}
}
// Función para calcular la transposición de una matriz
void transponerMatriz(int matriz[][MAX_COLUMNAS], int transpuesta[][MAX_COLUMNAS]) {
for (int i = 0; i < MAX_FILAS; i++) {
for (int j = 0; j < MAX_COLUMNAS; j++) {
transpuesta[j][i] = matriz[i][j];
}
}
}
// Función para calcular el determinante de una matriz 3x3
int calcularDeterminante(int matriz[][MAX_COLUMNAS]) {
return (matriz[0][0] * matriz[1][1] * matriz[2][2] +
matriz[0][1] * matriz[1][2] * matriz[2][0] +
matriz[0][2] * matriz[1][0] * matriz[2][1]) -
(matriz[0][2] * matriz[1][1] * matriz[2][0] +
matriz[0][0] * matriz[1][2] * matriz[2][1] +
matriz[0][1] * matriz[1][0] * matriz[2][2]);
}
// Función para imprimir una matriz
void imprimirMatriz(int matriz[][MAX_COLUMNAS]) {
for (int i = 0; i < MAX_FILAS; i++) {
for (int j = 0; j < MAX_COLUMNAS; j++) {
cout << matriz[i][j] << " ";
}
cout << endl;
}
}
int main() {
int opcion;
int matriz1[MAX_FILAS][MAX_COLUMNAS];
int matriz2[MAX_FILAS][MAX_COLUMNAS];
int resultadoSuma[MAX_FILAS][MAX_COLUMNAS];
int transpuesta[MAX_FILAS][MAX_COLUMNAS];
int determinante;
// Solicitar al usuario ingresar valores para las matrices
cout << "Ingrese los valores de la matriz 1 (fila por fila):" << endl;
for (int i = 0; i < MAX_FILAS; i++) {
for (int j = 0; j < MAX_COLUMNAS; j++) {
cin >> matriz1[i][j];
}
}
cout << "Ingrese los valores de la matriz 2 (fila por fila):" << endl;
for (int i = 0; i < MAX_FILAS; i++) {
for (int j = 0; j < MAX_COLUMNAS; j++) {
cin >> matriz2[i][j];
}
}
// Solicitar al usuario que elija una opción
cout << "Seleccione una opcion:" << endl;
cout << "1. Suma de matrices" << endl;
cout << "2. Transposicion de matriz" << endl;
cout << "3. Determinante de una matriz" << endl;
cin >> opcion;
switch (opcion) {
case 1:
// Suma de matrices
sumarMatrices(matriz1, matriz2, resultadoSuma);
cout << "Resultado de la suma de matrices:" << endl;
imprimirMatriz(resultadoSuma);
break;
case 2:
// Transposición de matriz
transponerMatriz(matriz1, transpuesta);
cout << "Transposicion de la matriz 1:" << endl;
imprimirMatriz(transpuesta);
break;
case 3:
// Determinante de una matriz
determinante = calcularDeterminante(matriz1);
cout << "Determinante de la matriz 1: " << determinante << endl;
break;
default:
cout << "Opcion invalida. Por favor seleccione 1, 2 o 3." << endl;
break;
}
return 0;
}
Tarea hecha en clase
bottom of page